概述
使用语句insert()
可以向图集的一个schema插入新的点和边。
// 插入点
insert().into(<schema>).nodes([
{<property1?>: <value1?>, <property2?>: <value2?>, ...},
{<property1?>: <value1?>, <property2?>: <value2?>, ...},
...
])
// 插入边
insert().into(<schema>).edges([
{_from: <fromValue>, _to: <toValue>, <property1?>: <value1?>, <property2?>: <value2?>, ...},
{_from: <fromValue>, _to: <toValue>, <property1?>: <value1?>, <property2?>: <value2?>, ...},
...
])
方法 |
参数 |
描述 |
---|---|---|
into() |
<schema> |
指定点schema或边schema,如@user |
nodes() 或edges() |
属性规范列表 | 将一或多个点或边插入指定schema,其中每个属性规范需用{} 包裹 |
属性规范列表中出现的每个属性都会被赋予给定的值,而缺失的自定义属性将默认赋值为null
。
请注意:
- 点的
_id
值若未指定,将由系统自动生成;也可根据需要为其指定唯一值。 - 点和边的
_uuid
值由系统自动生成,不允许指定。 - 必须提供边属性
_from
和_to
以指明边的起点和终点。
示例图集
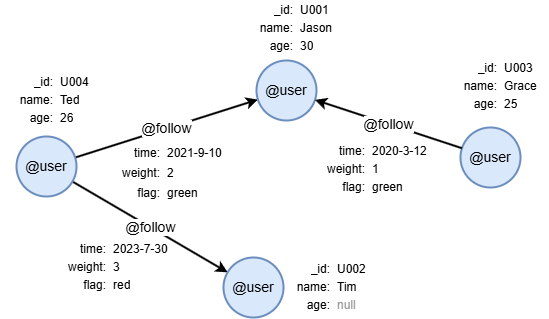
在一个空图集中,逐行运行以下UQL语句,创建示例图集:
create().node_schema("user").edge_schema("follow")
create().node_property(@user, "name").node_property(@user, "age", int32).node_property(@user, "location", point).node_property(@user, "isBlocked", bool).node_property(@user, "permissionCodes", "set(uint32)").edge_property(@follow, "time", datetime)
insert().into(@user).nodes([{_id:"U001", name:"Jason", age:30}, {_id:"U002", name:"Tim"}, {_id:"U003", name:"Grace", age:25}, {_id:"U004", name:"Ted", age:26}])
insert().into(@follow).edges([{_from:"U004", _to:"U001", time:"2021-9-10"}, {_from:"U003", _to:"U001", time:"2020-3-12"}, {_from:"U004", _to:"U002", time:"2023-7-30"}])
插入单个点/边
插入一个@user
点:
insert().into(@user).nodes({_id: "U005", name: "Alice"})
插入一条@follow
边:
insert().into(@follow).edges({_from: "U002", _to: "U001", time: "2023-8-9"})
插入单个点或边时,可省略
nodes()
或edges()
中的外层方括号[]
。
插入多个点/边
插入三个@user
点:
insert().into(@user).nodes([
{_id: "U006", name: "Lee", age: 12},
{name: "Alex"},
{}
])
插入两条@follow
边:
insert().into(@follow).edges([
{_from: "U001", _to: "U002"},
{_from: "U004", _to: "U003", time: "2023-9-10"}
])
返回插入的数据
插入两条@follow
边并返回插入边的所有信息:
insert().into(@follow).edges([
{_from: "U001", _to: "U004"},
{_from: "U001", _to: "U003", time: "2022-2-7"}
]) as e
return e{*}
结果:e
_uuid |
_from |
_to |
_from_uuid |
_to_uuid |
schema |
values |
---|---|---|---|---|---|---|
Sys-gen | U001 | U004 | UUID of U001 | UUID of U004 | follow | {time: null } |
Sys-gen | U001 | U003 | UUID of U001 | UUID of U003 | follow | {time: "2022-02-07 00:00:00"} |
插入布尔类型值
bool
类型的属性只接受1
(或true
)或0
(或false
)两个值。
如果赋除
0
以外的正整数,都自动转换成1
;赋其他值的结果无法预期。
插入一个@user
点,其属性isBlocked
的数据类型为bool
:
insert().into(@user).nodes({isBlocked: 0})
插入Point类型值
使用point()
函数为point
类型属性指定属性值。
插入一个@user
点,其属性location
的数据类型为point
:
insert().into(@user).nodes([
{location: point({latitude: 132.1, longitude: -1.5})}
]) as n
return n.location
结果:
n.location |
---|
POINT(132.1 -1.5) |
插入列表类型值
插入一个@user
点,其属性interests
的数据类型为string[]
:
insert().into(@user).nodes([
{interests: ["violin", "tennis", "movie"]}
])
插入集合类型值
插入一个@user
点,其属性permissionCodes
的数据类型为set(uint32)
:
insert().into(@user).nodes([
{permissionCodes: [1002, 1002, 1003, 1004]}
]) as n
return n.permissionCodes
结果:
n.permissionCodes |
---|
[1003,1004,1002] |
集合内的元素值会自动去重。