本节为您介绍如何使用Connection
对象的方法向图集插入或从图集删除点和边。
每个示例主要展示如何使用所列方法。点击完整示例查看完整代码示例。
每个示例主要展示如何使用所列方法。点击完整示例.
图数据模型示例
以下各示例为您展示如何在具有以下schema和属性的图集里插入或删除点和边:
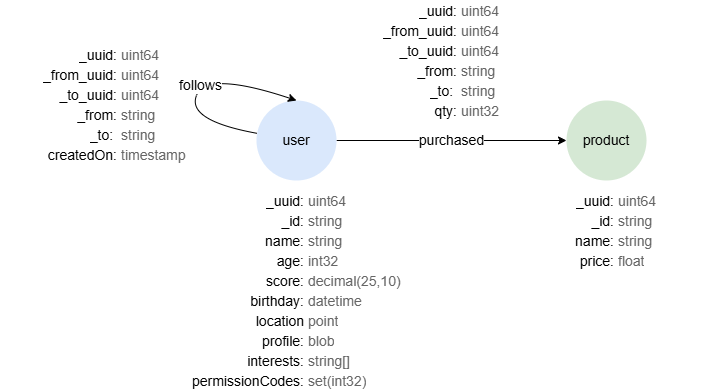
数据属性类型映射关系
插入点或边时,需指定不同属性类型。嬴图属性类型和C#/驱动程序数据类型的映射关系如下:
嬴图支持的属性类型 | C#/驱动程序支持的数据类型 |
---|---|
int32 | int |
uint32 | uint |
int64 | long |
uint64 | ulong |
float | float |
double | double |
decimal | Decimal (驱动程序类型) |
string | String |
text | String |
datetime | UltipaDatetime (驱动程序类型) |
timestamp | UltipaDatetime (驱动程序类型) |
point | Point (驱动程序类型) |
blob | byte[] 和 String |
list | List |
set | HashSet |
插入
InsertNodes()
向当前图集的某个schema插入新的点。
参数:
string
:schema名称List<Node>
:待插入的Node
列表。InsertRequestConfig
(可选):配置请求。
返回值:
UqlResponse
:请求的结果。若将InsertRequestConfig.silent
设定为false,Response
对象会包含一个nodes
别名,所有插入的点均在其中。
InsertRequestConfig insertRequestConfig = new InsertRequestConfig()
{
InsertType = InsertType.Normal,
Graph = "lcc",
Silent = false,
};
// 向图集lcc名为user的schema插入两个点,打印错误代码和插入点的信息
var myPoint = new Point();
myPoint.X = 23.63M;
myPoint.Y = 104.25M;
Node node1 = new Node()
{
Uuid = 1,
Id = "U001",
Values = new()
{
{ "name", "Alice" },
{ "age", 18 },
{ "score", 65.32M },
{ "birthday", new DateTime(1993, 5, 4) },
{ "location", myPoint },
{ "profile", "abc" },
{
"interests",
new List<string>() { "tennis", "violin" }
},
{
"permissionCodes",
new HashSet<int> { 2004, 3025, 1025 }
},
},
};
Node node2 = new Node()
{
Uuid = 2,
Id = "U002",
Values = new() { { "name", "Bob" } },
};
List<Node> nodeList = new List<Node>();
nodeList.Add(node1);
nodeList.Add(node2);
var res = await ultipa.InsertNodes("user", nodeList, insertRequestConfig);
if (res.Status.ErrorCode == 0)
{
Console.WriteLine("Insertion succeeds");
}
// 如果InsertRequestConfig.silent设定为true,Response不返回别名
List<Node> insertedNodes = res?.Alias("nodes")?.AsNodes();
foreach (var node in insertedNodes)
{
Console.WriteLine(JsonConvert.SerializeObject(node));
}
Insertion succeeds
{"Uuid":1,"Id":"U001","Schema":"user","Values":{"name":"Alice","age":18,"score":65.3200000000,"birthday":"1993-05-04T00:00:00Z","location":{"X":23.630000,"Y":104.250000},"profile":"YWJj","interests":["tennis","violin"],"permissionCodes":null}}
{"Uuid":2,"Id":"U002","Schema":"user","Values":{"name":"Bob","age":null,"score":null,"birthday":null,"location":null,"profile":null,"interests":null,"permissionCodes":null}}
InsertEdges()
向当前图集的某个schema插入新的边。
参数:
string
:schema名称List<Edge>
:待插入的Edge
列表。InsertRequestConfig
(可选):配置请求。
返回值:
UqlResponse
:请求的结果。若将InsertRequestConfig.silent
设定为false,ResponseInsertEdge
对象会包含一个edges
别名,所有插入的边均在其中。
InsertRequestConfig insertRequestConfig = new InsertRequestConfig()
{
InsertType = InsertType.Normal,
Graph = "lcc",
Silent = false,
};
// 向图集lcc名为follows的schema插入两条边,打印错误代码和插入边的信息
List<Edge> edgeList = new List<Edge>();
Edge edge1 = new Edge()
{
Uuid = 1,
FromId = "U001",
ToId = "U002",
Values = new() { { "createdOn", new DateTime(2024, 5, 6) } },
};
Edge edge2 = new Edge()
{
Uuid = 2,
FromId = "U002",
ToId = "U001",
Values = new() { { "createdOn", new DateTime(2024, 5, 8) } },
};
edgeList.Add(edge1);
edgeList.Add(edge2);
var res = await ultipa.InsertEdges("follows", edgeList, insertRequestConfig);
if (res.Status.ErrorCode == 0)
{
Console.WriteLine("Insertion succeeds");
}
// 如果InsertRequestConfig.silent为true,则Response中没有别名
List<Edge> insertedEdges = res?.Alias("edges")?.AsEdges();
foreach (var edge in insertedEdges)
{
Console.WriteLine(JsonConvert.SerializeObject(edge));
}
Insertion succeeds
{"Uuid":1,"FromUuid":1,"ToUuid":2,"Id":"","FromId":"U001","ToId":"U002","Schema":"follows","Values":{"createdOn":"2024-05-05T16:00:00Z"}}
{"Uuid":2,"FromUuid":2,"ToUuid":1,"Id":"","FromId":"U002","ToId":"U001","Schema":"follows","Values":{"createdOn":"2024-05-07T16:00:00Z"}}
InsertNodesBatchBySchema()
通过gRPC向当前图集的某个schema插入新点。插入的点,其属性必须和schema结构中的声明保持一致。
参数:
Schema
:目标schema。List<Node>
:待插入的Node
列表。InsertRequestConfig
(可选):配置请求。
返回值:
InsertResponse
:请求的结果。
// 向图集lcc名为user的schema插入两个点,打印错误代码和插入结果
InsertRequestConfig insertRequestConfig = new InsertRequestConfig()
{
InsertType = InsertType.Normal,
Graph = "lcc",
Silent = false,
};
List<Property> properties = new List<Property>();
Property property1 = new Property() { Name = "name", Type = PropertyType.String };
Property property2 = new Property() { Name = "age", Type = PropertyType.Int32 };
Property property3 = new Property() { Name = "score", Type = PropertyType.Decimal };
Property property4 = new Property() { Name = "birthday", Type = PropertyType.Datetime };
Property property5 = new Property() { Name = "location", Type = PropertyType.Point };
Property property6 = new Property() { Name = "profile", Type = PropertyType.Blob };
Property property7 = new Property()
{
Name = "interests",
Type = PropertyType.List,
SubTypes = new PropertyType[] { PropertyType.String },
};
Property property8 = new Property()
{
Name = "permissionCodes",
Type = PropertyType.Set,
SubTypes = new PropertyType[] { PropertyType.Int32 },
};
properties.Add(property1);
properties.Add(property2);
properties.Add(property3);
properties.Add(property4);
properties.Add(property5);
properties.Add(property6);
properties.Add(property7);
properties.Add(property8);
Schema schema = new Schema()
{
Name = "user",
Properties = properties,
DbType = DBType.Dbnode,
};
var myPoint = new Point();
myPoint.X = 23.63M;
myPoint.Y = 104.25M;
Node node1 = new Node()
{
Uuid = 1,
Id = "U001",
Values = new()
{
{ "name", "Alice" },
{ "age", 18 },
{ "score", 65.32M },
{ "birthday", new DateTime(1993, 5, 4) },
{ "location", myPoint },
{ "profile", new byte[] { 123 } },
{
"interests",
new List<string> { "tennis", "violin" }
},
{
"permissionCodes",
new HashSet<int> { 2004, 3025, 1025 }
},
},
};
Node node2 = new Node()
{
Uuid = 2,
Id = "U002",
Values = new() { { "name", "Bob" } },
};
List<Node> nodeList = new List<Node>();
nodeList.Add(node1);
nodeList.Add(node2);
var res = ultipa.InsertNodesBatchBySchema(schema, nodeList, insertRequestConfig);
Console.WriteLine(res.Status.ErrorCode);
Console.WriteLine(JsonConvert.SerializeObject(res));
Success
{"Status":{"ErrorCode":0,"Msg":"","ClusterInfo":null},"Statistic":{"NodeAffected":0,"EdgeAffected":0,"TotalCost":0,"EngineCost":0},"Data":{"Uuids":[1,2],"Ids":[],"ErrorItem":{}}}
InsertEdgesBatchBySchema()
通过gRPC向当前图集的某个schema插入新边。插入的边,其属性必须和schema结构中的声明保持一致。
参数:
Schema
:目标schema。List<Edge>
:待插入的Edge
列表。InsertRequestConfig
(可选):配置请求。
返回值:
InsertResponse
:请求的结果。
// 向图集lcc名为follows的schema插入两条边,打印错误代码和插入结果
InsertRequestConfig insertRequestConfig = new InsertRequestConfig()
{
InsertType = InsertType.Normal,
Graph = "lcc",
Silent = false,
};
List<Property> properties = new List<Property>();
Property property = new Property() { Name = "createdOn", Type = PropertyType.Datetime };
properties.Add(property);
Schema schema = new Schema() { Name = "follows", Properties = properties };
List<Edge> edgeList = new List<Edge>();
Edge edge1 = new Edge()
{
Uuid = 1,
FromId = "U001",
ToId = "U002",
Values = new() { { "createdOn", new DateTime(2024, 5, 6) } },
};
Edge edge2 = new Edge()
{
Uuid = 2,
FromId = "U002",
ToId = "U001",
Values = new() { { "createdOn", new DateTime(2024, 5, 8) } },
};
edgeList.Add(edge1);
edgeList.Add(edge2);
var res = ultipa.InsertEdgesBatchBySchema(schema, edgeList, insertRequestConfig);
Console.WriteLine(res.Status.ErrorCode);
Console.WriteLine(JsonConvert.SerializeObject(res.Data));
Success
{"Uuids":[1,2],"Ids":[],"ErrorItem":{}}
InsertNodesBatchAuto()
通过gRPC向当前图集的一个或多个schema插入新点。插入的点,其属性必须和schema结构中的声明保持一致。
参数:
List<Node>
:待插入的Node
列表。InsertRequestConfig
(可选):配置请求。
返回值:
Dictionary<string,InsertResponse>
:请求的结果。
// 向图集lcc名为user和product的两个schema各插入一个点,打印错误代码和插入结果
InsertRequestConfig insertRequestConfig = new InsertRequestConfig()
{
InsertType = InsertType.Normal,
Graph = "lcc",
Silent = false,
};
var myPoint = new Point();
myPoint.X = 23.63M;
myPoint.Y = 104.25M;
Node node1 = new Node()
{
Schema = "user",
Uuid = 1,
Id = "U001",
Values = new()
{
{ "name", "Alice" },
{ "age", 18 },
{ "score", 65.32M },
{ "birthday", new DateTime(1993, 5, 4) },
{ "location", myPoint },
{ "profile", new byte[] { 123 } },
{
"interests",
new List<string>() { "tennis", "violin" }
},
{
"permissionCodes",
new HashSet<int> { 2004, 3025, 1025 }
},
},
};
Node node2 = new Node()
{
Schema = "user",
Uuid = 2,
Id = "U002",
Values = new() { { "name", "Bob" } },
};
Node node3 = new Node()
{
Schema = "product",
Uuid = 3,
Id = "P001",
Values = new() { { "name", "Wireless Earbud" }, { "price", 93.2F } },
};
List<Node> nodeList = new List<Node>();
nodeList.Add(node1);
nodeList.Add(node2);
nodeList.Add(node3);
var res = await ultipa.InsertNodesBatchAuto(nodeList, insertRequestConfig);
Console.WriteLine(JsonConvert.SerializeObject(res));
{"user":{"Status":{"ErrorCode":0,"Msg":"","ClusterInfo":null},"Statistic":{"NodeAffected":0,"EdgeAffected":0,"TotalCost":0,"EngineCost":0},"Data":{"Uuids":[1,2],"Ids":[],"ErrorItem":{}}},"product":{"Status":{"ErrorCode":1,"Msg":"Duplicated ids!","ClusterInfo":null},"Statistic":{"NodeAffected":0,"EdgeAffected":0,"TotalCost":0,"EngineCost":0},"Data":{"Uuids":[],"Ids":[],"ErrorItem":{}}}}
InsertEdgesBatchAuto()
通过gRPC向当前图集的一个或多个schema插入新边。插入的边,其属性必须和schema结构中的声明保持一致。
参数:
List<Edge>
:待插入的Edge
列表。InsertRequestConfig
(可选):配置请求。
返回值:
Dictionary<string,InsertResponse>
:请求的结果。
// 向图集lcc名为follows的schema插入两条边,向名为purchased的schema插入一条边,打印错误代码
InsertRequestConfig insertRequestConfig = new InsertRequestConfig()
{
InsertType = InsertType.Normal,
Graph = "lcc",
Silent = false,
};
List<Edge> edgeList = new List<Edge>();
Edge edge1 = new Edge()
{
Schema = "follows",
Uuid = 1,
FromId = "U001",
ToId = "U002",
Values = new() { { "createdOn", new DateTime(2024, 5, 6) } },
};
Edge edge2 = new Edge()
{
Schema = "follows",
Uuid = 2,
FromId = "U002",
ToId = "U001",
Values = new() { { "createdOn", new DateTime(2024, 5, 8) } },
};
Edge edge3 = new Edge()
{
Schema = "purchased",
Uuid = 3,
FromId = "U002",
ToId = "P001",
Values = new() { { "qty", 1 } },
};
edgeList.Add(edge1);
edgeList.Add(edge2);
edgeList.Add(edge3);
var res = await ultipa.InsertEdgesBatchAuto(edgeList, insertRequestConfig);
Console.WriteLine(JsonConvert.SerializeObject(res));
{"follows":{"Status":{"ErrorCode":0,"Msg":"insert edges succeed!","ClusterInfo":null},"Statistic":{"NodeAffected":0,"EdgeAffected":0,"TotalCost":0,"EngineCost":0},"Data":{"Uuids":[1,2],"Ids":[],"ErrorItem":{}}},"purchased":{"Status":{"ErrorCode":0,"Msg":"insert edges succeed!","ClusterInfo":null},"Statistic":{"NodeAffected":0,"EdgeAffected":0,"TotalCost":0,"EngineCost":0},"Data":{"Uuids":[3],"Ids":[],"ErrorItem":{}}}}
删除
DeleteNodes()
从图集删除符合指定条件的点。需特别注意,删除点的同时也会删除与点相连的边。
参数:
string
:待删除的点的条件。InsertRequestConfig
(可选):配置请求。
返回值:
<UqlResponse, List<Node>?>
:请求的结果。若将InsertRequestConfig.Slient
设定为false,Response
对象会包含一个nodes
别名,所有删除的点均在其中。
// 删除图集lcc里@user下名为Alice的点,打印错误代码
// 与删除的点相连的边也会全部删除
InsertRequestConfig insertRequestConfig = new InsertRequestConfig()
{
InsertType = InsertType.Normal,
Graph = "lcc",
Silent = false,
};
var res = await ultipa.DeleteNodes("@user.name == 'Alice'", insertRequestConfig);
Console.WriteLine(res.Item1.Status.ErrorCode);
Console.WriteLine(JsonConvert.SerializeObject(res.Item2));
Success
[{"Uuid":1,"Id":"U001","Schema":"user","Values":{}}]
DeleteEdges()
从图集删除符合指定条件的边。
参数:
string
:用于指定要删除边的过滤条件。InsertRequestConfig
(可选):配置请求。
返回值:
<UqlResponse, List<Edge>?>
:请求的结果。若将InsertRequestConfig.Slient
设定为false,Response
对象会包含一个edges
别名,所有删除的边均在其中。
// 从图集lcc删除@purchased中的全部边,打印错误代码
InsertRequestConfig insertRequestConfig = new InsertRequestConfig()
{
InsertType = InsertType.Normal,
Graph = "lcc",
Silent = false,
};
var res = await ultipa.DeleteEdges("@purchased", insertRequestConfig);
Console.WriteLine(res.Item1.Status.ErrorCode);
Console.WriteLine(JsonConvert.SerializeObject(res.Item2));
Success
[{"Uuid":3,"FromUuid":2,"ToUuid":3,"Id":"","FromId":"U002","ToId":"P001","Schema":"purchased","Values":{}}]
完整示例
using System.Data;
using System.Security.Cryptography.X509Certificates;
using System.Xml.Linq;
using Google.Protobuf.WellKnownTypes;
using Microsoft.Extensions.Logging;
using Newtonsoft.Json;
using UltipaService;
using UltipaSharp;
using UltipaSharp.api;
using UltipaSharp.configuration;
using UltipaSharp.connection;
using UltipaSharp.exceptions;
using UltipaSharp.structs;
using UltipaSharp.utils;
using Logger = UltipaSharp.utils.Logger;
using Property = UltipaSharp.structs.Property;
using Schema = UltipaSharp.structs.Schema;
class Program
{
static async Task Main(string[] args)
{
// 设置连接
//URI 示例: Hosts=new[]{"mqj4zouys.us-east-1.cloud.ultipa.com:60010"}
var myconfig = new UltipaConfig()
{
Hosts = new[] { "192.168.1.85:60061", "192.168.1.86:60061", "192.168.1.87:60061" },
Username = "***",
Password = "***",
};
// 建立与数据库的连接
var ultipa = new Ultipa(myconfig);
var isSuccess = ultipa.Test();
Console.WriteLine(isSuccess);
// 配置插入请求
InsertRequestConfig insertRequestConfig = new InsertRequestConfig()
{
InsertType = InsertType.Normal,
Graph = "lcc",
Silent = false,
};
// 向图集lcc名为user的schema插入两个点,向名为product的schema插入一个点,打印错误代码和插入结果
var myPoint = new Point();
myPoint.X = 23.63M;
myPoint.Y = 104.25M;
Node node1 = new Node()
{
Schema = "user",
Uuid = 1,
Id = "U001",
Values = new()
{
{ "name", "Alice" },
{ "age", 18 },
{ "score", 65.32M },
{ "birthday", new DateTime(1993, 5, 4) },
{ "location", myPoint },
{ "profile", new byte[] { 123 } },
{
"interests",
new List<string>() { "tennis", "violin" }
},
{
"permissionCodes",
new HashSet<int> { 2004, 3025, 1025 }
},
},
};
Node node2 = new Node()
{
Schema = "user",
Uuid = 2,
Id = "U002",
Values = new() { { "name", "Bob" } },
};
Node node3 = new Node()
{
Schema = "product",
Uuid = 3,
Id = "P001",
Values = new() { { "name", "Wireless Earbud" }, { "price", 93.2F } },
};
List<Node> nodeList = new List<Node>();
nodeList.Add(node1);
nodeList.Add(node2);
nodeList.Add(node3);
var res = await ultipa.InsertNodesBatchAuto(nodeList, insertRequestConfig);
Console.WriteLine(JsonConvert.SerializeObject(res));
}
}