本节介绍用于插入点、边的方法。
图结构
本节示例的点、边插入和删除基于如下的Schema和属性定义进行:
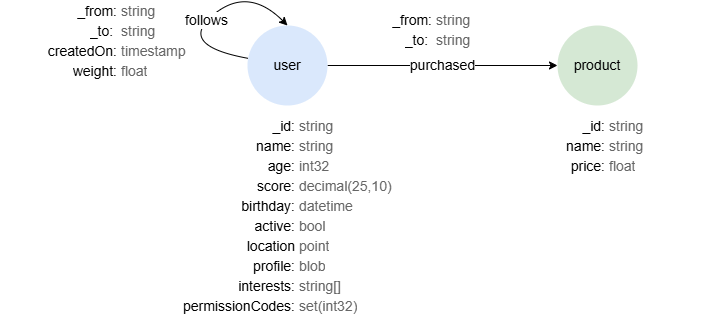
insertNodes()
向图中某个Schema插入点。
参数
nodes:List[Node]
:待插入的点列表;每个Node
的uuid
属性不允许设置。schemaName: str
:Schema名称。InsertRequestConfig
(可选):请求配置。
返回值
Response
:请求结果。
# Inserts two 'user' nodes into the graph 'social'
insertRequestConfig = InsertRequestConfig(graph="social")
nodes = [
Node(id="U1", values={
"name": "Alice",
"age": 18,
"score": 65.32,
"birthday": "1993-5-4",
"active": 0,
"location": "point({latitude: 132.1, longitude: -1.5})",
"profile": "castToRaw(abc)",
"interests": ["tennis", "violin"],
"permissionCodes": [2004, 3025, 1025]
}),
Node(id="U2", values={
"name": "Bob"
})
]
response = Conn.insertNodes(nodes, "user", insertRequestConfig)
if response.status.code.name == "SUCCESS":
print(response.status.code.name)
else:
print(response.status.message)
SUCCESS
insertEdges()
向图中某个Schema插入边。
参数
edges:List[Edge]
:待插入的边列表;每个Edge
的fromId
和toId
属性必填,uuid
、fromUuid
和toUuid
不允许设置。schemaName: str
:Schema名称。InsertRequestConfig
(可选):请求配置。
返回值
Response
:请求结果。
# Inserts two 'follows' edges to the graph 'social'
insertRequestConfig = InsertRequestConfig(graph="social")
edges = [
Edge(fromId="U1", toId="U2", values={"createdOn": "2024-5-6", "weight": 3.2}),
Edge(fromId="U2", toId="U1", values={"createdOn": 1715169600})
]
response = Conn.insertEdges(edges, "follows", insertRequestConfig)
if response.status.code.name == "SUCCESS":
print(response.status.code.name)
else:
print(response.status.message)
SUCCESS
insertNodesBatchBySchema()
通过gRPC向图中某个Schema插入点。此方法针对批量插入进行了优化,要求每个Node.values
与定义的Schema.properties
结构相同。
参数
schema: Schema
:目标Schema;属性name
和dbType
必填,properties
包含部分或所有属性。nodes:List[Node]
:待插入的点列表;每个Node
的uuid
属性不允许设置,values
必须与Schema.properties
结构相同。InsertRequestConfig
(可选):请求配置。
返回值
InsertResponse
:插入请求结果。
# Inserts two 'user' nodes into the graph 'social'
insertRequestConfig = InsertRequestConfig(graph="social")
schema = Schema(
name="user",
dbType=DBType.DBNODE,
properties=[
Property(name="name", type=UltipaPropertyType.STRING),
Property(name="age", type=UltipaPropertyType.INT32),
Property(name="score", type=UltipaPropertyType.DECIMAL),
Property(name="birthday", type=UltipaPropertyType.DATETIME),
Property(name="active", type=UltipaPropertyType.BOOL),
Property(name="location", type=UltipaPropertyType.POINT),
Property(name="profile", type=UltipaPropertyType.BLOB),
Property(name="interests", type=UltipaPropertyType.LIST, subType=[UltipaPropertyType.STRING]),
Property(name="permissionCodes", type=UltipaPropertyType.SET, subType=[UltipaPropertyType.INT32])
]
)
nodes = [
Node(id="U1", values={
"name": "Alice",
"age": 18,
"score": 65.32,
"birthday": "1993-5-4",
"active": 0,
"location": "point({latitude: 132.1, longitude: -1.5})",
"profile": "castToRaw(abc)",
"interests": ["tennis", "violin"],
"permissionCodes": [2004, 3025, 1025]
}),
Node(id="U2", values={
"name": "Bob",
"age": None,
"score": None,
"birthday": None,
"active": None,
"location": None,
"profile": None,
"interests": None,
"permissionCodes": None
})
]
insertResponse = Conn.insertNodesBatchBySchema(schema, nodes, insertRequestConfig)
if insertResponse.errorItems:
print("Error items:", insertResponse.errorItems)
else:
print("All nodes inserted successfully")
All nodes inserted successfully
insertEdgesBatchBySchema()
通过gRPC向图中某个Schema插入边。此方法针对批量插入进行了优化,要求每个Edge.values
与定义的Schema.properties
结构相同。
参数
schema: Schema
:目标Schema;属性name
和dbType
必填,properties
包含部分或所有属性。edges:List[Edge]
:待插入的边列表;每个Edge
的fromId
和toId
属性必填,uuid
、fromUuid
和toUuid
不允许设置,values
必须与Schema.properties
结构相同。InsertRequestConfig
(可选):请求配置。
返回值
InsertResponse
:插入请求结果。
# Inserts two 'follows' edges into the graph 'social'
insertRequestConfig = InsertRequestConfig(graph="social")
schema = Schema(
name="follows",
dbType=DBType.DBEDGE,
properties=[
Property(name="createdOn", type=UltipaPropertyType.TIMESTAMP),
Property(name="weight", type=UltipaPropertyType.FLOAT)
]
)
edges = [
Edge(fromId="U1", toId="U2", values={"createdOn": "2024-5-6", "weight": 3.2}),
Edge(fromId="U2", toId="U1", values={"createdOn": 1715169600, "weight": None})
]
insertResponse = Conn.insertEdgesBatchBySchema(schema, edges, insertRequestConfig)
if insertResponse.errorItems:
print("Error items:", insertResponse.errorItems)
else:
print("All edges inserted successfully")
All edges inserted successfully
insertNodesBatchAuto()
通过gRPC向图中一个或多个Schema插入点。此方法针对批量插入进行了优化,要求相同schema
的Node
有相同的values
结构。
参数
nodes:List[Node]
:待插入的点列表;每个Node
的uuid
属性不允许设置,schema
必填,schema
相同的Node
有相同的values
结构。InsertRequestConfig
(可选):请求配置。
返回值
Dict[str,InsertResponse]
:Schema名称,插入请求结果。
# Inserts two 'user' nodes and a 'product' node into the graph 'social'
insertRequestConfig = InsertRequestConfig(graph="social")
nodes = [
Node(id="U1", schema="user", values={
"name": "Alice",
#"age": 18,
"score": 65.32,
"birthday": "1993-5-4",
"active": True,
"location": "point({latitude: 132.1, longitude: -1.5})",
"profile": "castToRaw(abc)",
"interests": ["tennis", "violin"],
"permissionCodes": [2004, 3025, 1025]
}),
Node(id="U2", schema="user", values={
"name": "Bob",
#"age": None,
"score": None,
"birthday": None,
"active": None,
"location": None,
"profile": None,
"interests": None,
"permissionCodes": None
}),
Node(schema="product", values={
"name": "Wireless Earbud",
"price": 93.2
})
]
result = Conn.insertNodesBatchAuto(nodes, insertRequestConfig)
for schemaName, insertResponse in result.items():
if insertResponse.errorItems:
print("Error items of", schemaName, "nodes:", insertResponse.errorItems)
else:
print("All", schemaName, "nodes inserted successfully")
All user nodes inserted successfully
All product nodes inserted successfully
insertEdgesBatchAuto()
通过gRPC向图中一个或多个Schema插入边。此方法针对批量插入进行了优化,要求相同schema
的Edge
有相同的values
结构。
参数
edges:List[Edge]
:待插入的边列表;每个Edge
的fromId
和toId
属性必填,uuid
、fromUuid
和toUuid
不允许设置,schema
必填,schema
相同的Edge
有相同的values
结构。InsertRequestConfig
(可选):请求配置。
返回值
Dict[str,InsertResponse]
:Schema名称,插入请求结果。
# Inserts two 'follows' edges and a 'purchased' edge into the graph 'social'
insertRequestConfig = InsertRequestConfig(graph="social")
edges = [
Edge(schema="follows", fromId="U1", toId="U2", values={"createdOn": "2024-05-06", "weight": 3.2}),
Edge(schema="follows", fromId="U2", toId="U1", values={"createdOn": 1715169600, "weight": None}),
Edge(schema="purchased", fromId="U2", toId="67da1ae90000020020000013", values={})
]
result = Conn.insertEdgesBatchAuto(edges, insertRequestConfig)
for schemaName, insertResponse in result.items():
if insertResponse.errorItems:
print("Error items of", schemaName, "edges:", insertResponse.errorItems)
else:
print("All", schemaName, "edges inserted successfully")
All follows edges inserted successfully
All purchased edges inserted successfully
完整示例
from ultipa import UltipaConfig, Connection, InsertRequestConfig, Node
ultipaConfig = UltipaConfig()
# URI example: ultipaConfig.hosts = ["https://mqj4zouys.us-east-1.cloud.ultipa.com:60010"]
ultipaConfig.hosts = ["192.168.1.85:60061", "192.168.1.87:60061", "192.168.1.88:60061"]
ultipaConfig.username = "<username>"
ultipaConfig.password = "<password>"
Conn = Connection.NewConnection(defaultConfig=ultipaConfig)
# Inserts two 'user' nodes, a 'product' node, two 'follows' edges, and a 'purchased' edge into the graph 'social'
insertRequestConfig = InsertRequestConfig(graph="social")
nodes = [
Node(id="U1", schema="user", values={
"name": "Alice",
#"age": 18,
"score": 65.32,
"birthday": "1993-5-4",
"active": True,
"location": "point({latitude: 132.1, longitude: -1.5})",
"profile": "castToRaw(abc)",
"interests": ["tennis", "violin"],
"permissionCodes": [2004, 3025, 1025]
}),
Node(id="U2", schema="user", values={
"name": "Bob",
#"age": None,
"score": None,
"birthday": None,
"active": None,
"location": None,
"profile": None,
"interests": None,
"permissionCodes": None
}),
Node(id="P1", schema="product", values={
"name": "Wireless Earbud",
"price": 93.2
})
]
edges = [
Edge(schema="follows", fromId="U1", toId="U2", values={"createdOn": "2024-05-06", "weight": 3.2}),
Edge(schema="follows", fromId="U2", toId="U1", values={"createdOn": 1715169600, "weight": None}),
Edge(schema="purchased", fromId="U2", toId="P1", values={})
]
result_n = Conn.insertNodesBatchAuto(nodes, insertRequestConfig)
for schemaName, insertResponse in result_n.items():
if insertResponse.errorItems:
print("Error items of", schemaName, "nodes:", insertResponse.errorItems)
else:
print("All", schemaName, "nodes inserted successfully")
result_e = Conn.insertEdgesBatchAuto(edges, insertRequestConfig)
for schemaName, insertResponse in result_e.items():
if insertResponse.errorItems:
print("Error items of", schemaName, "edges:", insertResponse.errorItems)
else:
print("All", schemaName, "edges inserted successfully")